Build Twig Components with Storybook
A modern way of building, maintaining and documenting your visual driven twig components in storybook with an automated and opinionated integration workflow for your CMS.
1. Define your Design Tokens
Everything starts with well defined Design Tokens.
Collect all your spaces colors typography from your design before you start coding components. Good Design Tokens are a big timesaver!
2.1. Build your UI Patterns
When you are done with your design tokens you can continue with building your patterns. Start with your smallest components first, followed by the larger ones.
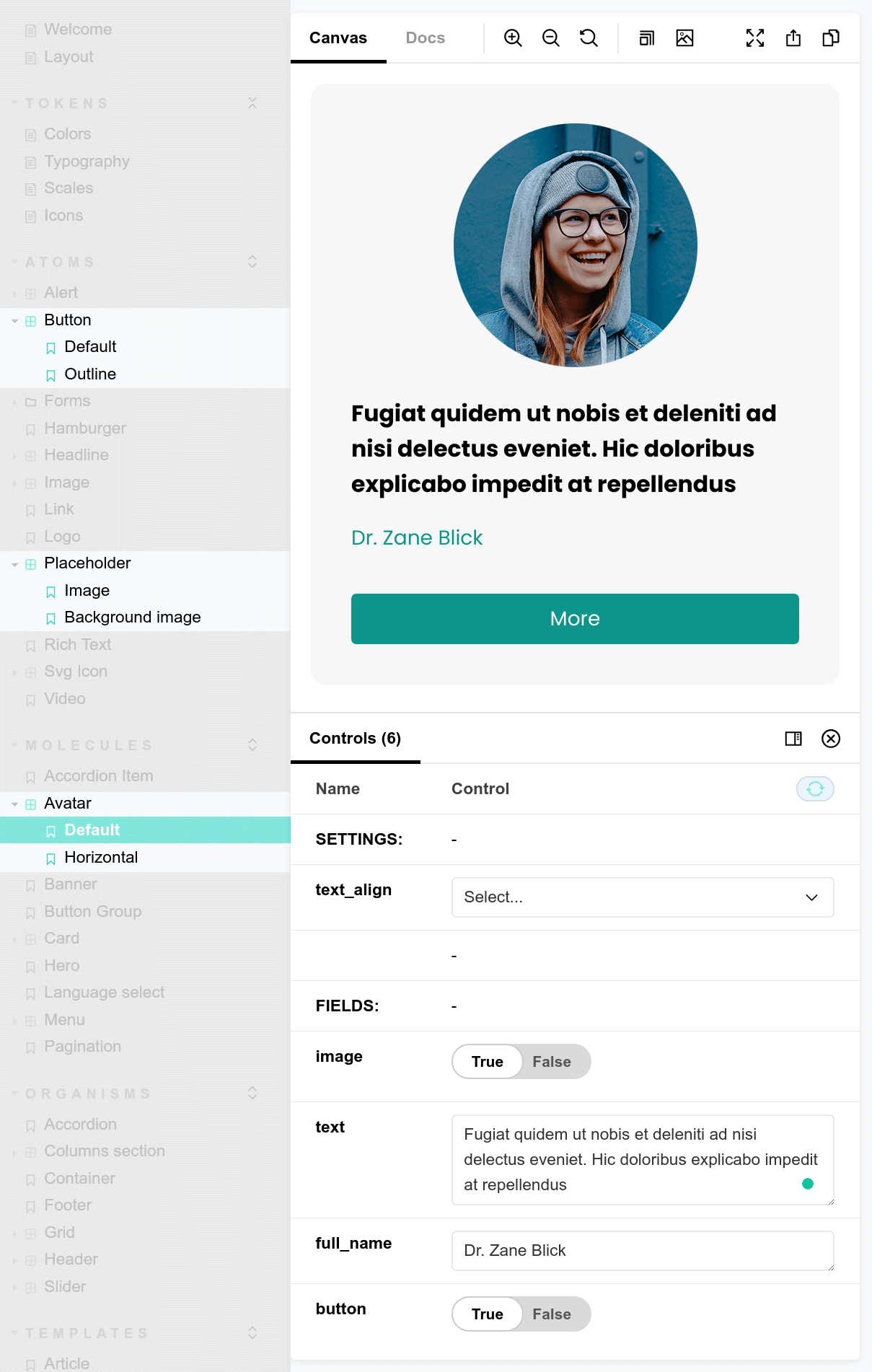
2.2. Document your components
3. Make Love.
After finalizing your component, you can easily use it in other apps like Drupal.
Try it now!
Get started using the automated command line tool. This command creates a Wingsuit demo project. The demo page uses the atomic design principle to structure the patterns..
You might also try ...Drupal Kickstarter
Wingsuit Kickstarter is the fastest way to start your Drupal project with Wingsuit. The Kickstarter uses UI Patterns to map Patterns to Drupal and Acquia Blt for automation.